Building a Weather Forecaster tool using Python and OpenWeatherMap API
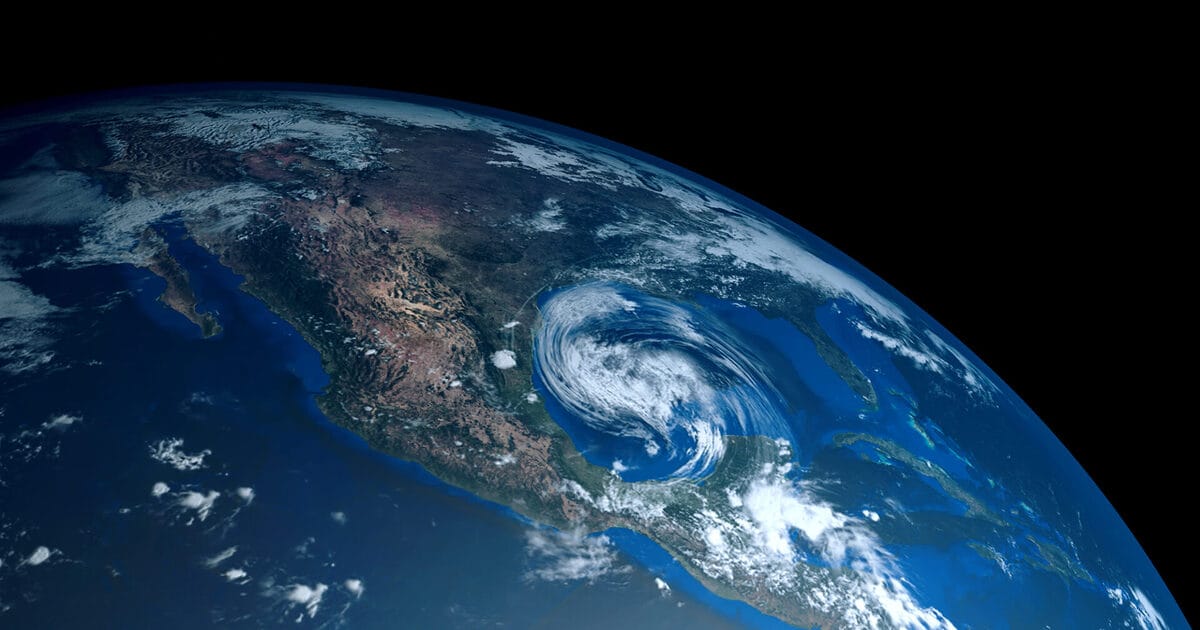
Hi, everyone, In this blog post I will walk you through the process of building a simple weather forecast tool using Python and the OpenWeatherMap API.
By the end of this project we'll have a handy script that can provide us with the current temperature and weather description for any city around the world. To build this project, it is essential to have python installed in the system, an API key from OpenWeatherMap which can be obtained free by signing up account (https://home.openweathermap.org/users/sign_up)
First, Lets import requests module.This particular module allows us to made http requests to the server. If you don't have this module, you can install this using command
pip3 install requests
Here is the final python script
while True:
import requests
api_key = 'ddcf42916c446c8b49f079abc7ed4c29'
city = input('\nEnter city name: ')
url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
temp = data['main']['temp']
desc = data['weather'][0]['description']
##Converting Tempt to clesius from kelvin.
print(f' \nTemperature in clesius:{round(temp - 273.15,2)}C')
print(f'Temperature in fahrenheit:{round(temp * 9/5 - 459.67,2) } F')
print(f'Description: {desc}\n')
print ("Hit CTRL C to exit")
else:
print('Error fetching weather data, Exitting')
exit()
Explanation
- This script starts by prompting the user to enter a city name.
- It constructs a URL with the city name and the API key, then makes a GET request to the OpenWeatherMap API.
- If the request is successful (status code 200), it parses the JSON response to extract the temperature and weather description.
- The temperature is converted from Kelvin to Celsius and Fahrenheit before printing.
- If there's an error fetching the data, the script exits with an error message.
So, well we're now equipped with the knowledge to build our own weather forecast tool and stay informed about the weather conditions no matter where we are!
Thanks for reading my blog. STAY UPDATED ;)